This article will explain what is heap implementation in Python. We will learn the difference between max-heap and min-heap and how max-heap and min-heap are used in Python programs.
What is Heap?
A heap is a data structure that satisfies the heap property and adheres to the properties of a complete binary tree. As a result, it is also known as a binary heap.
The complete binary tree is, as everyone knows, a tree in which each level is filled and all nodes are as far to the left as possible. It is possible for the last level of the binary tree to be devoid of information.
You’re probably wondering what the heap property is at this point. Each node in the heap data structure is assigned a key-value pair or weight. The key value of the root node is compared to that of the children’s nodes, and the tree is then divided into max-heap and min-heap groups. A heap data structure is basically a method for sorting the items in an array or list called “heapsort.”
Max-heap and min-heap are the two types of heap data structures. Before we look at max-heap and min-heap, we’ll look at heapify, which will help us understand max-heap and min-heap.
Additionally, heaps are binary trees for which every parent node has a value less than or equal to any of its children. This implementation uses arrays for which heap[k] <= heap[2*k+1]
and heap[k] <= heap[2*k+2]
for all k, counting elements from zero. Based on Python Official Documentation.
What is Heapify?
Heapify refers to the operation of generating a heap data structure from a binary tree. The heapify
operation is used to construct the Max-Heap and the Min-Heap. Examine the Heapify with the help of the following example.
Take a look at the input array in the figure below:
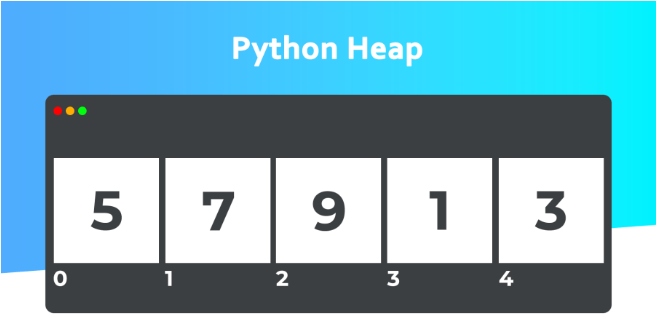
We will make the whole binary tree using this array:
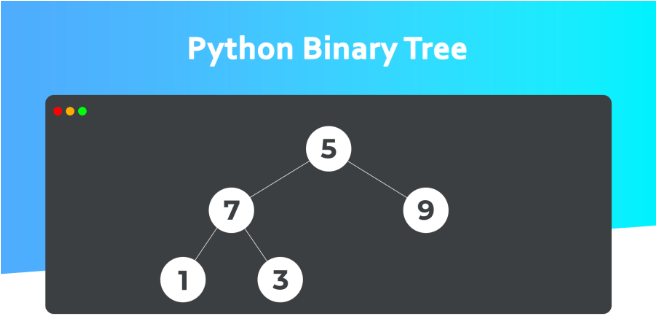
We will start the heapify process as follows:
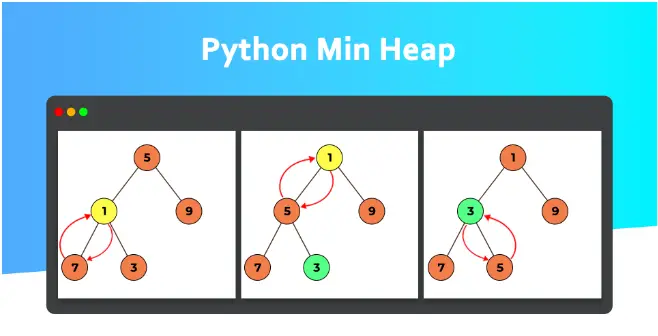
The default process of heapify
is min heap. So, the above illustration shows the step-by-step process of making a min heap.
The value of each internal node is less than the value of its child node in a Min heap. The process of min heap is shown in the picture above.
We all know that the leftmost child node of 7 has the lowest value, which is 1. The root of the binary tree must be that child node. To do this, we need to swap the nodes one by one until the node with the lowest value is the root node.
Once the lowest value is on the root node, we have to look at the other parent and child nodes to see if other parents are lower than their child nodes. Remember that the rule for the min heap is that each internal node’s value must be less than that of its child.
So, as you can see, 5 is bigger than 3, so 3 and 5 need to be switched.
So the array output of Min Heap is:
[1, 3, 9, 7, 5]
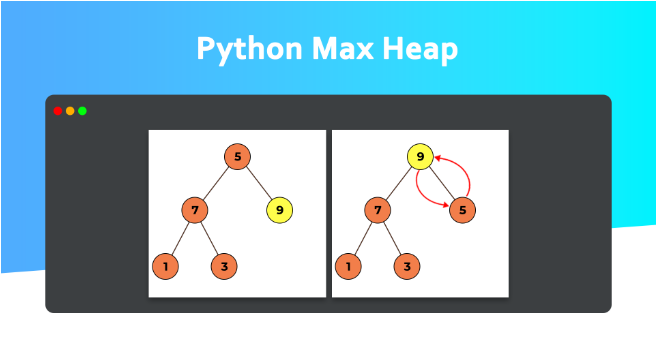
The max heap, on the other hand, is the opposite of the min heap. The max heap is the value of each internal node that is greater than or equal to the value of its children node.
The child node of 5 on the right is the largest value among all the nodes in the binary tree, which is the value 9. Once we identify the largest value in the binary tree, then we can start swapping the 9 and the 5.
So the array output of Max Heap is:
[9, 7, 5, 1, 3]
Min Heap vs Max Heap
The following is the difference between Min Heap and Max Heap in Python:
Min Heap | Max Heap |
---|---|
The key of the parent node is smaller than or equal to that of the child node. | The key of the parent node is larger than or equal to that of the child node. |
The root node is the minimum key element. | The root node is the maximum key element. |
Uses the ascending priority | Uses the descending priority |
When building the min heap, the smallest node comes first. | When building the max heap, the largest node comes first. |
The smallest elements are popped out of the heap | The largest element is popped out of the heap |
Frequently Ask Questions
Is there a heap in Python?
Yes, there is a heap in Python. To use the heap in Python, we must import the heapq
module.
Is Python heap Min or Max?
A heap is a unique tree structure in which each parent node is smaller or equal to its child node. Then the heap is referred to as a min heap. If every parent node is greater than or equal to its child node, then the heap is referred to as a max heap.
Summary
When working with graphs or trees, the heap data structure is a very useful data structure. The heap data structure helps us make our programs and problem statements work better.
We can use min-heap and max-heap to process data sets because they work well. As soon as you know how to use the heap data structure well, you are ready to work with graphs and trees at a higher level.
Lastly, if you want to learn more about Heap implementation Python, please leave a comment below. We’ll be happy to hear it!