Are you ready to unfold and learn all about what is event bubbling and event capturing in JavaScript?
In this comprehensive article, you’ll understand how they work, their differences, and their importance in web development.
There are two ways events can move through the elements in a web page in JavaScript.
When an event happens on an element like a, button click, it doesn’t just stop there. It can also be handled by its parent elements.
This whole process is called event propagation. The two mechanisms for this are known as event bubbling and event capturing.
What is event bubbling in JavaScript?
Event bubbling is a term used in JavaScript to describe the behavior of events in the DOM (Document Object Model).
Here’s the sample code:
<!DOCTYPE html>
<html>
<head>
<style>
body {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
</style>
</head>
<body>
<h2 style="color:blue">Welcome to Itsourcecode</h2>
<div>
<button>Click Me!</button>
</div>
</body>
</body>
</html>
Output:
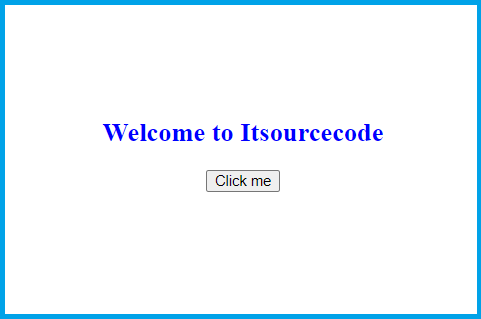
When an event is triggered on an element, it doesn’t just affect that element.
Instead, the event “bubbles up” through the element’s ancestors in the DOM tree, triggering the same event on each one until it reaches the root element.
It simply means that if you have a button inside a div, and you click on the button, not only will the button’s click event be triggered, but so will the div’s click event.
Here’s the illustration
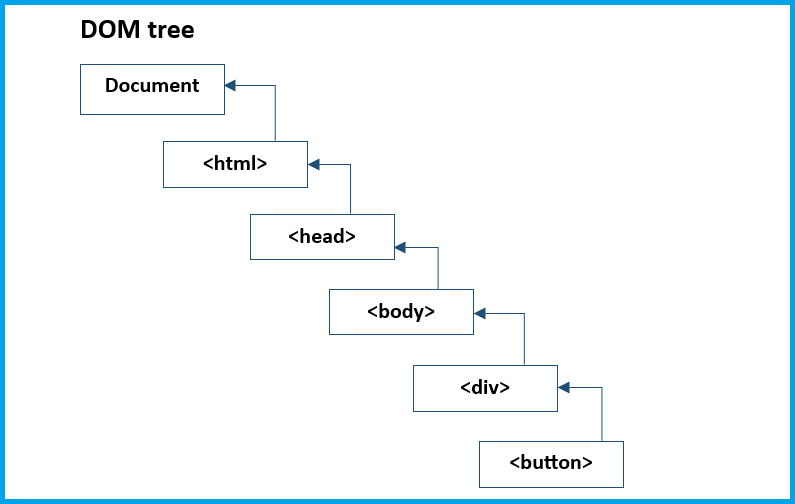
This behavior can be useful when you want to handle an event on a parent element instead of on the actual element that received the event. This is known as event delegation.
In most browsers, event bubbling is the usual way events are handled.
When an event takes place on an element inside another element (for example, a button inside a div), the event is first dealt with by the innermost element and then passed up through its parent elements in the DOM tree, until it reaches the highest level, which is the document level.
Here’s the Illustration showing how event bubbling works:
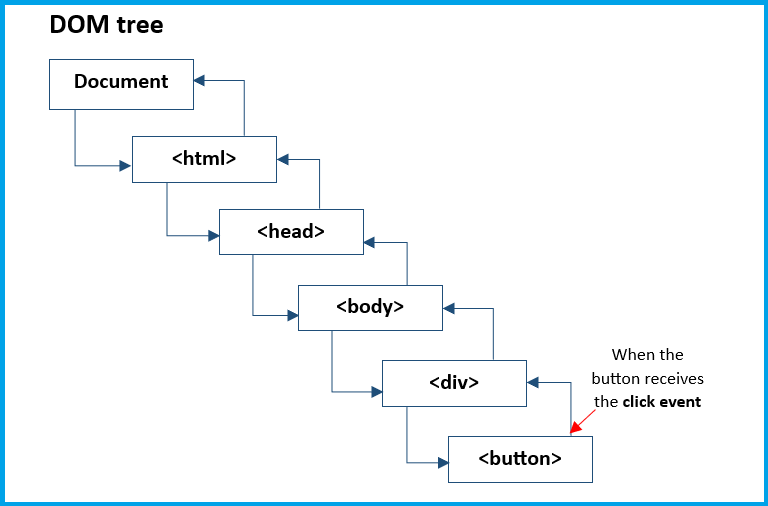
What is event capturing in JavaScript?
Event capturing also known as event trickling is the opposite of event bubbling.
It is a concept in the DOM (Document Object Model) where an event triggered on an element is first captured by the outermost ancestor element and then propagated down to the target element through its ancestors.
It simply means that when an event occurs on an element, it triggers the event on the target element’s ancestors first before reaching the target element.
The event handlers on the ancestor elements will be triggered first, followed by the target element’s event handler
Here’s the illustration:
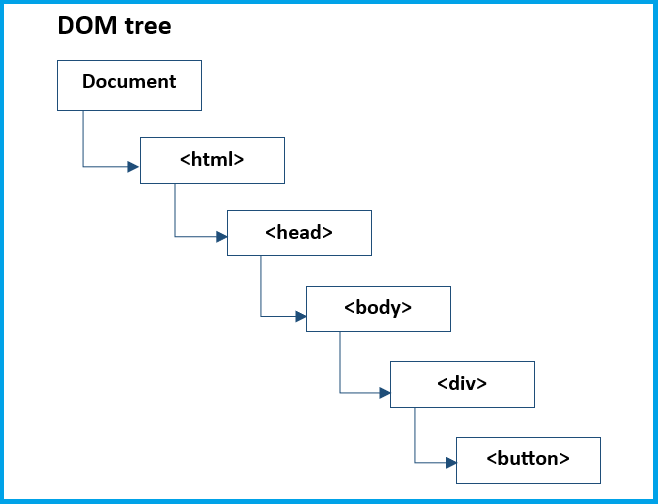
Difference between event bubbling and event capturing in JavaScript
Event bubbling and capturing are two ways of event propagation in the HTML DOM API, when an event occurs in an element inside another element, and both elements have registered a handle for that event.
The event propagation mode determines in which order the elements receive the event. With bubbling, the event is first captured and handled by the innermost element and then propagated to outer elements.
With capturing, the event is first captured by the outermost element and propagated to the inner elements.
Capturing, also known as ‘trickling,’ aids in recalling the propagation order: trickling down and bubbling up.
Here’s the illustration to see the difference between event bubbling and event capturing:
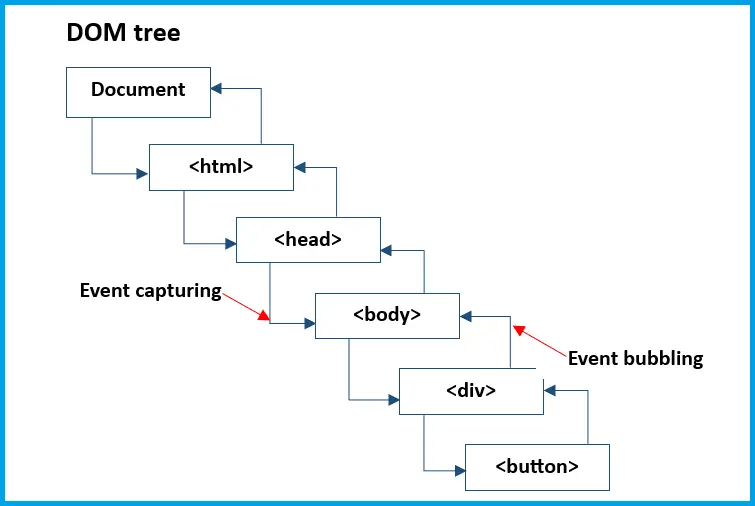
If you click on <button>, then the sequence is:
Event capturing phase, the first listener:
DOCUMENT→ HTML →HEAD→ BODY → DIV → BUTTON
Event capturing phase, the first listener:
BUTTON → DIV → BODY → HEAD→ HTML → DOCUMENT
Moreover, event bubbling is a technique where the event handlers are invoked when one element is nested into the other element and part of the same event.
We can understand event bubbling as a sequence of calling the event handlers when one element is nested in another element, and both elements have registered listeners for the same event.
So beginning from the deepest element to its parents covering all its ancestors on the way to top to bottom, calling is performed.
On the other hand, event capturing is the opposite of event bubbling, as we mentioned above.
In event capturing, the flow goes from the outermost element to the target element.
Whereas, in the case of event bubbling, the flow goes from the target element to the outermost element.
How to stop event bubbling in JavaScript?
In order to stop the event from bubbling up to parent elements, you can use the stopPropagation method of the event object.
Here’s an example:
button.addEventListener("click", (event) => {
event.stopPropagation(); ✅
// do anything with the event object
});
In our example, when the button is clicked, the click event handler is called. Inside the handler, we call the stopPropagation method of the event object.
This will stop the event from bubbling up to parent elements and prevent any parent handlers from being notified of the event.
Conclusion
In conclusion, this article discusses event bubbling and event capturing, which are the two mechanisms in JavaScript used for event propagation in the DOM.
Event bubbling allows an event to propagate from the innermost element to its ancestors, triggering the same event on each ancestor until it reaches the root element.
Meanwhile, event capturing propagates the event from the outermost ancestor to the target element. These two mechanisms offer different ways to handle events in the DOM.
Aside from that, we illustrate how to stop an event from bubbling up to parent elements. The stopPropagation() method of the event object can be used.
We are hoping that this article provides you with enough information that helps you understand event bubbling and event capturing in JavaScript.
You can also check out the following article:
Thank you for reading itsourcecoders 😊.