Are you ready to learn about JavaScript multidimensional arrays with clear explanations and practical examples?
JavaScript multidimensional arrays are a powerful tool for organizing and manipulating complex data in your programs.
With clear explanations and practical examples, this guide will help you understand how to create, access, and manipulate arrays of arrays in JavaScript.
What is a multidimensional array JavaScript?
A multidimensional array is an array of arrays in JavaScript. In other words, it is an array where each element is also an array.
It is used to store data in a tabular form, where each element is defined by multiple indices.
JavaScript does not provide the multidimensional array natively, but you can create one by defining an array of elements, where each element is also another array.
How to create multidimensional array in JavaScript?
In JavaScript, you can create a multidimensional array by defining an array of elements, where each element is also another array.
Example 1:
Here’s an example of how to create a two-dimensional array:
let sampleArray = [
[10, 20],
[30, 40],
[50, 60]
];
In this example, sampleArray is a two-dimensional array with three rows and two columns.
The first row contains the elements 10 and 20, the second row contains the elements 30 and 40, and the third row contains the elements 50 and 60.
How to access multidimensional array in JavaScript? You can access the elements of a multidimensional array using the square bracket notation.
For example, to access the element in the first row and second column of sampleArray, you can use the following code:
let element = sampleArray[0][1];
console.log(element);
Output:
20
You can also use loops to iterate over the elements of a multidimensional array.
Here’s an example that shows how to use a nested for loop to iterate over all the elements of sampleArray:
for (let i = 0; i < sampleArray.length; i++) {
for (let j = 0; j < sampleArray[i].length; j++) {
console.log(sampleArray[i][j]);
}
}
This code will output all the elements of sampleArray in row-major order.
Output:
10
20
30
40
50
60
Example 2:
let employee1 = ["It", 18];
let employee2 = ["Source", 19];
let employee3 = ["Code", 20];
let employeesData = [employee1, employee2, employee3];
console.log(employeesData);
This code creates three arrays, employee1, employee2, and employee3, each representing a employee with their name and age.
Then, it creates a two-dimensional array named employeesData that stores the information about all three employees.
When you run this code, you’ll see the following output:
[ [ 'It', 18 ], [ 'Source', 19 ], [ 'Code', 20 ] ]
This shows the information about all three students stored in the employeesData array.
How to declare multidimensional array in JavaScript?
To declare or define a multidimensional array with more than two dimensions by nesting arrays within arrays.
Here’s an example on how you can declare a three-dimensional array:
let sampleArray = [
[
[1, 2],
[3, 4]
],
[
[5, 6],
[7, 8]
]
];
let element = sampleArray[0][1];
console.log(element);
In this example, sampleArray is a three-dimensional array with two layers, two rows, and two columns.
Output:
[ 3, 4 ]
What is an example of a multidimensional array?
Here’s an example of a JavaScript code that uses a multidimensional array to store and manipulate data about different subjects and the number of hours spent studying each subject per day:
let subjects = [
['Math', 2],
['Science', 2],
['English', 1],
['History', 1],
['Programming', 1]
];
console.log('Original array:');
console.table(subjects);
// Add a new element to the array
subjects.push(['Web Development',1]);
console.log('After adding a new element:');
console.table(subjects);
// Remove the last element from the array
subjects.pop();
console.log('After removing the last element:');
console.table(subjects);
// Accessing elements of the array
console.log('First subject: ' + subjects[0][0]);
console.log('Hours spent on first subject: ' + subjects[0][1]);
As you can see in our example code, we create a two-dimensional array named subjects that stores information about different subjects and the number of hours spent studying each subject per day.
The program then uses various Array methods such as push(), pop(), and table() to manipulate and display the elements of the array.
When you run this program, you’ll see the following output:
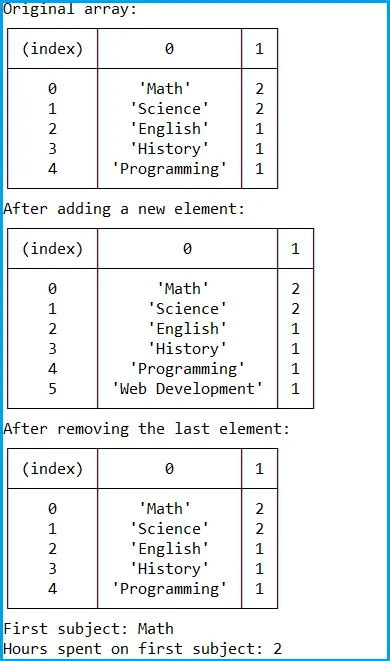
Adding an Element to the array
Adding elements to a multidimensional array can be accomplished by employing either the Array’s push() method or using indexing notation.
let subjects = [
['Math', 2],
['Science', 2],
['English', 1],
['History', 1],
['Programming', 1]
];
subjects.push(['Web Development',1]);
console.log('After adding a new element:');
console.table(subjects);
After adding a new element, here’s the output:
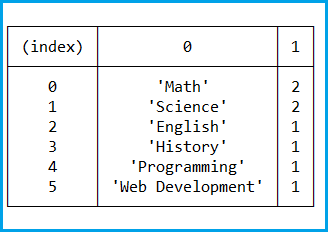
Remove the last element from the array
The removal of elements from inner arrays can be achieved by utilizing the pop() method, which can also be applied to eliminate entire inner arrays.
let subjects = [
['Math', 2],
['Science', 2],
['English', 1],
['History', 1],
['Programming', 1],
['We Development', 1]
];
subjects.pop();
console.log('After removing the last element:');
console.table(subjects);
After removing the last element, here’s the output:
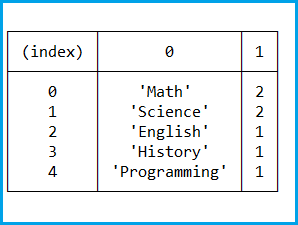
How to iterate over the elements of a JavaScript multidimensional array?
Here’s an example of how to iterate over the elements of a JavaScript multidimensional array:
let subjects = [
['Math', 2],
['Science', 2],
['English', 1],
['History', 1],
['Programming', 1],
['Web Development', 1]
];
for (let i = 0; i < subjects.length; i++) {
console.log('Subject: ' + subjects[i][0] + ', Hours: ' + subjects[i][1]);
}
This code uses a for loop to iterate over the rows of the subjects array.
Inside the loop, it uses the i variable to access the current row of the array and then logs the subject name and the number of hours spent studying that subject to the console.
When you run this code, you’ll see the following output:
let subjects = [
['Math', 2],
['Science', 2],
['English', 1],
['History', 1],
['Programming', 1],
['Web Development', 1]
];
for (let i = 0; i < subjects.length; i++) {
console.log('Subject: ' + subjects[i][0] + ', Hours: ' + subjects[i][1]);
}
This shows the information about all the subjects stored in the subjects array.
Conclusion
In conclusion, this article explains in detail the JavaScript Multidimensional Array.
A multidimensional array is an array of arrays where each element is also an array.
It allows you to store data in a structured way, with multiple dimensions representing different aspects of the data.
For example, you could use a two-dimensional array to represent a matrix or a table of data.
In this article, you learn how to create multidimensional arrays in JavaScript and how to access their elements using the square bracket notation.
You also discover how to use loops and other techniques to iterate over the elements of a multidimensional array and manipulate them in various ways.
We are hoping that this article provides you with enough information that helps you understand the JavaScript multidimensional array.
You can also check out the following article:
Thank you for reading itsourcecoders 😊.