JavaScript is a powerful programming language that has revolutionized web development. One of its key features is the ability to control the appearance of web elements dynamically. In this article, we’ll dive into the fascinating world of “JavaScript background color.”
We’ll explore how to change background colors on your website using JavaScript, understand the importance of color in user experience, and discover practical tips to make your website visually appealing.
So, let’s get started!
What is Javascript background color?
The background color of an element refers to the hue that fills its area on a web page.
By leveraging JavaScript, you can dynamically alter these hues to create visually striking effects and improve the overall user experience.
How to Change JavaScript Background Color?
Changing the background color with JavaScript is an essential skill for web developers. There are multiple ways to achieve this, each offering unique advantages.
Let’s explore these methods step-by-step:
Method 1: Using the document Object
The simplest way to change the background color is by manipulating the document
object. By accessing the document.body
property, you can modify the background color of the entire page.
const newBackgroundColor = "#ffcc00"; // Replace with your desired color code
document.body.style.backgroundColor = newBackgroundColor;
Method 2: Targeting Specific Elements
To change the background color of specific elements, such as headings or paragraphs, you can use their respective IDs or class names. Here’s an example of changing the background color of an element with a given ID:
<!DOCTYPE html>
<html>
<head>
<title>Change Background Color</title>
</head>
<body>
<h1 id="header">Welcome to ITsourcecode</h1>
<p id="paragraph">This is some sample text.</p>
<script>
const headerElement = document.getElementById("header");
const paragraphElement = document.getElementById("paragraph");
headerElement.style.backgroundColor = "blue";
paragraphElement.style.backgroundColor = "yellow";
</script>
</body>
</html>
Output:

Method 3: Responding to User Interaction
You can make your web pages more interactive by changing the background color in response to user actions, such as clicking a button. Here’s an example of using an onclick
event to change the background color:
<!DOCTYPE html>
<html>
<head>
<title>Change Background Color</title>
</head>
<body>
<button onclick="changeBackgroundColor()">Click Me</button>
<script>
function changeBackgroundColor() {
const bodyElement = document.body;
const randomColor = getRandomColor();
bodyElement.style.backgroundColor = randomColor;
}
function getRandomColor() {
const letters = "0123456789ABCDEF";
let color = "#";
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
</script>
</body>
</html>
Output:
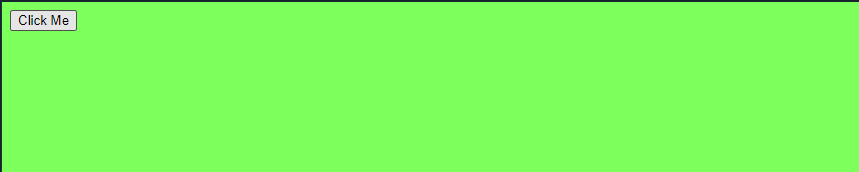
Method 4: Animating Background Color
Adding animation to background color changes can create stunning visual effects. You can achieve this using CSS transitions or JavaScript libraries like jQuery or GSAP (GreenSock Animation Platform).
<!DOCTYPE html>
<html>
<head>
<title>Change Background Color with Animation</title>
<style>
#box {
width: 100px;
height: 100px;
background-color: red;
transition: background-color 1s ease; /* CSS Transition */
}
</style>
</head>
<body>
<div id="box"></div>
<script>
const boxElement = document.getElementById("box");
function changeColorWithAnimation() {
const randomColor = getRandomColor();
boxElement.style.backgroundColor = randomColor;
}
function getRandomColor() {
const letters = "0123456789ABCDEF";
let color = "#";
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
setInterval(changeColorWithAnimation, 2000); // Change color every 2 seconds
</script>
</body>
</html>
Output:
How to Change JavaScript Background Color of Specific Elements?
Changing the background color of specific elements allows you to focus on highlighting certain parts of your web page.
Here are some methods to target specific elements effectively:
Using getElementById Method:
The getElementById method is one of the most commonly used ways to select specific elements by their unique IDs and modify their background color.
const element = document.getElementById("elementID");
element.style.backgroundColor = "#ff0000"; // Replace with your desired color code
Using getElementsByClassName Method:
If you have multiple elements with the same class name and want to change their background color, you can use the getElementsByClassName method.
const elements = document.getElementsByClassName("className");
for (let i = 0; i < elements.length; i++) {
elements[i].style.backgroundColor = "#00ff00"; // Replace with your desired color code
}
Using getElementsByTagName Method:
If you wish to change the background color of all elements of a specific tag, such as <p>
or <h2>
, you can use the getElementsByTagName method.
const paragraphs = document.getElementsByTagName("p");
for (let i = 0; i < paragraphs.length; i++) {
paragraphs[i].style.backgroundColor = "#0000ff"; // Replace with your desired color code
}
Using querySelector and querySelectorAll Methods:
The querySelector and querySelectorAll methods allow you to select elements using CSS selectors.
You can change the background color of elements that match the selector.
const firstParagraph = document.querySelector("p");
firstParagraph.style.backgroundColor = "#ff00ff"; // Replace with your desired color code
const allHeaders = document.querySelectorAll("h2");
allHeaders.forEach(header => {
header.style.backgroundColor = "#ffcc00"; // Replace with your desired color code
});
How to Change JavaScript Background Color Dynamically?
Dynamically changing the background color of elements based on user interactions or other events can make your website more engaging.
Here are some examples of dynamic background color changes:
Example 1: Change Background Color on Button Click
You can use JavaScript to change the background color when a user clicks a button.
<!DOCTYPE html>
<html>
<head>
<title>Change Background Color on Button Click</title>
</head>
<body>
<button onclick="changeBackgroundColor()">Change Color</button>
<script>
function changeBackgroundColor() {
const body = document.body;
body.style.backgroundColor = getRandomColor();
}
function getRandomColor() {
const letters = "0123456789ABCDEF";
let color = "#";
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
</script>
</body>
</html>
Output:
Example 2: Change Background Color on Mouseover
You can change the background color when the user hovers over an element using the onmouseover
event.
<!DOCTYPE html>
<html>
<head>
<title>Change Background Color on Mouseover</title>
<style>
#box {
width: 100px;
height: 100px;
background-color: red;
}
</style>
</head>
<body>
<div id="box" onmouseover="changeBackgroundColor()"></div>
<script>
function changeBackgroundColor() {
const box = document.getElementById("box");
box.style.backgroundColor = getRandomColor();
}
function getRandomColor() {
const letters = "0123456789ABCDEF";
let color = "#";
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
</script>
</body>
</html>
Example 3: Change Background Color Based on Time
You can create a dynamic background that changes color based on the time of day.
<!DOCTYPE html>
<html>
<head>
<title>Change Background Color Based on Time</title>
</head>
<body>
<div id="greeting">Good Morning!</div>
<script>
function changeBackgroundColor() {
const greeting = document.getElementById("greeting");
const currentTime = new Date().getHours();
if (currentTime >= 5 && currentTime < 12) {
greeting.textContent = "Good Morning!";
document.body.style.backgroundColor = "#ffcc00"; // Morning color
} else if (currentTime >= 12 && currentTime < 18) {
greeting.textContent = "Good Afternoon!";
document.body.style.backgroundColor = "#00ff00"; // Afternoon color
} else {
greeting.textContent = "Good Evening!";
document.body.style.backgroundColor = "#0000ff"; // Evening color
}
}
</script>
</body>
</html>
Nevertheless, here are other functions you can learn to enhance your JavaScript skills.
- How to Dynamically Add Rows in HTML table using JavaScript?
- What is JavaScript insertion sort? Explained How It Works
- What is rest parameters JavaScript?
Conclusion
Congratulations! You’ve now mastered the art of changing the background color using JavaScript. From the basics of manipulating the document object to dynamic color changes based on user interactions, you have the tools to create visually stunning web pages. Remember to experiment with different methods and unleash your creativity to design captivating user experiences.