TypeScript offers a bunch of handy string methods that make it easy for developers to work with strings.
You can search, replace, trim, and change the case of strings, among other things.
These tools help you tackle all sorts of string-related tasks, making your code more efficient and easy to manage.
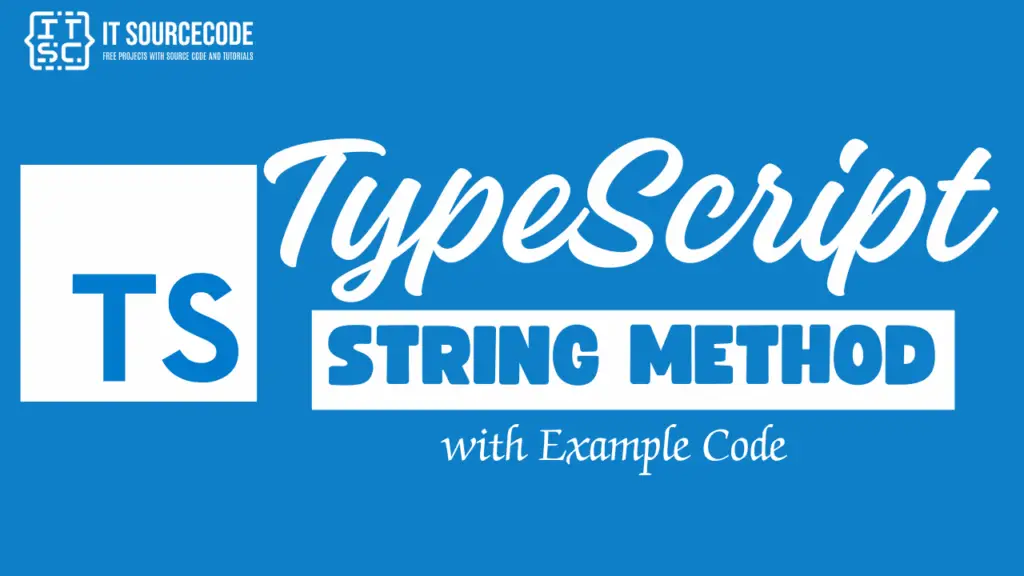
TypeScript String Methods
Here are some of the string methods in TypeScript with example code:
1. charAt()
The charAt() method returns the character at a specified index.
let SampleText = "Itsourcecode".charAt(1); ✅
console.log(SampleText);
Output:
t
2. charCodeAt()
The charCodeAt() method returns the Unicode value of the character at a specified index.
let Sample = "Itsourcecode".charCodeAt(1); ✅
console.log(Sample);
Output:
116
3. concat()
The concat() method combines two or more strings.
let Sample = "It".concat(" ", "SourceCode"); ✅
console.log(Sample);
Output:
It SourceCode
4. indexOf()
The indexOf() method returns the index of the first occurrence of a specified substring.
let Sample = "Hi, welcome to Itsourcecode".indexOf("Itsourcecode"); ✅
console.log(Sample);
Output:
15
5. lastIndexOf()
The lastIndexOf() method returns the index of the last occurrence of a specified substring.
let Sample = "Hi, welcome to Itsourcecode".lastIndexOf("e"); ✅
console.log(Sample);
Output:
26
6. replace()
The replace() method replaces a specified substring with another substring.
let Sample = "It Source Coder".replace("Coder", "Code"); ✅
console.log(Sample);
Output:
It Source Code
7. split()
The split() method splits a string into an array of substrings.
let Sample = "It source code".split(" "); ✅
console.log(Sample);
Output:
[ 'It', 'source', 'code' ]
8. toUpperCase()
The toUpperCase() method converts all characters in a string to uppercase.
let Sample = "itsourcecode".toUpperCase(); ✅
console.log(Sample);
Output:
ITSOURCECODE
9. toLowerCase()
The toLowerCase() method converts all characters in a string to lowercase.
let Sample = "ItSourceCode".toLowerCase();
console.log(Sample);
Output:
itsourcecode
10. includes()
The includes() method checks if a string contains another string.
let Sample = "Hi Welcome to Itsourcecode".includes("Itsourcecode"); ✅
console.log(Sample);
Output:
true
11. startsWith()
The startsWith() method checks if a string starts with another string.
let Sample = "Hi Welcome to Itsourcecode".startsWith("Hi"); ✅
console.log(Sample);
Output:
true
12. endsWith()
The endsWith() method checks if a string ends with another string.
let Sample = "Hi Welcome to Itsourcecode".endsWith("Itsourcecode"); ✅
console.log(Sample);
Output:
true
13. slice()
The slice() method Extracts a section of a string and returns it as a new string.
let Sample = "Hi Welcome to Itsourcecode".slice(14, 26); ✅
console.log(Sample);
Output:
Itsourcecode
14. substring()
The substring() method returns a substring between two specified indices.
let Sample = "Hi Welcome to Itsourcecode".substring(0, 10); ✅
console.log(Sample);
Output:
Hi Welcome
15. trim()
The trim() method eliminates whitespace from a string in both ends.
let Sample = " Hi Welcome to Itsourcecode ".trim(); ✅
console.log(Sample);
Output:
Hi Welcome to Itsourcecode
16. valueOf()
The valueOf() method returns the basic value of the given object.
let sample = new String("Hi Welcome to Itsourcecode!"); ✅
console.log(sample.valueOf());
Output:
Hi Welcome to Itsourcecode!
17. toString()
The toString() method returns a string that represents the given object.
let sample = 143;
console.log(sample.toString()); ✅
Output:
143
18. substr()
The substr() method returns a portion of the string, starting at the specified index and extending for a given number of characters.
let sample = "Itsourcecode";
console.log(sample.substr(0, 2)); ✅
console.log(sample.substr(2, 6)); ✅
console.log(sample.substr(8, 10)); ✅
Output:
It
source
code
19. search()
The search() method searches a string for a match against a regular expression and returns the index of the first match.
If no match is found, it returns -1.
let sample = "Hi Welcome to Itsourcecode!!";
let index = sample.search(/Itsourcecode/); ✅
console.log(index);
Output:
14
20. match()
The match() method retrieves the matches when matching a string against a regular expression.
let sample = "Item price is $100.99";
let matches = sample.match(/\$(\d+)(?:\.(\d+))?/); ✅
if (matches) {
console.log("Dollars:", matches[1]);
if (matches[2]) {
console.log("Cents:", matches[2]);
}
}
21. localeCompare()
The localeCompare() returns a number that shows if a reference string precedes, follows, or matches the given string in sorting order.
let sample1 = "It";
let sample2 = "Sourcecode";
console.log(sample1.localeCompare(sample2)); ✅
Output:
-1
22. toLocaleLowerCase()
The toLocaleLowerCase() method converts a string to lowercase letters, according to the host’s locale.
let Samplestring = "Welcome to Itsourcecode!";
let newstr = Samplestring.toLocaleLowerCase(); ✅
console.log(newstr);
Output:
welcome to itsourcecode!
23. toLocaleUpperCase()
The toLocaleUpperCase() method converts a string to uppercase letters, according to the host’s locale.
let Samplestring = "Welcome to Itsourcecode!";
var newstr = Samplestring.toLocaleUpperCase(); ✅
console.log(newstr);
Output:
WELCOME TO ITSOURCECODE!
Conclusion
So this is the end of our discussion about String Methods in TypeScript. TypeScript has a bunch of powerful string methods that make working with strings a breeze.
Whether you’re searching, replacing, trimming, or changing the case of your strings, these methods are super easy to understand and help keep your code efficient and easy to manage.
If you have any questions or inquiries, please don’t hesitate to leave a comment below.