CRUD Operation in PHP and MySQL with Source Code
CRUD in PHP – This article will teach you how to make CRUD Operations in PHP and MySQL with Source Code which is free to download. This CRUD System in PHP and PDO comes with examples and free source code that will help you create your own.
A CRUD Operation in PHP interacts with records in a table and is frequently used in connection with a database. In our CRUD System in PHP, we’ll be using MySQL as our database management system.
What is CRUD?
The abbreviation CRUD stands for Create, Read, Update, and Delete. Basic database data processing is known as CRUD operations. To conduct these activities, we can use a PHP program and a MySQL database.
What is PDO PHP extension?
PDO is an abbreviation for PHP Data Objects. PDO is a simple, consistent way of accessing databases. This simply means developers and programmers can design portable code much simpler.
CRUD Operation in PHP and MySQL with Source Code Table of contents
- Step 1. Getting Started for CRUD Operation in PHP
- Step 2. Crud Operations in PHP and MYSQL Database
- Step 3. Creating the CRUD Operations in PHP Stylesheet (CSS3)
- Step 4. Creating the CRUD Operations in PHP Page
- CRUD in PHP and MySQL with Source Code – Project Overview
- Download CRUD System in PHP Source Code Below
- Conclusion
- Inquiries
Step 1. Getting Started for CRUD Operation in PHP
We need to install our local web server and set up our app before we can start developing our CRUD System in PHP
1.1 What This Tutorial Will Teach You
- Create MySQL Records – Add new records to the table.
- Reading MySQL Records and displaying them in an HTML table.
- Update MySQL Records – In the Contacts table, update existing MySQL records.
- Delete MySQL Records — Confirm and delete Contacts table records.
- GET and POST Requests – Use an HTML form and URL parameters to send data to our program.
- Prepared Statements – Prepared statements let us protect our SQL statements.
1.2 Requirements
- Web Server – I recommend installing XAMPP on your local computer system since it contains MySQL, PHP, phpMyAdmin, and the PDO extension.
- PHP – The most recent version of PHP is recommended, although earlier versions should operate just fine (skip if you installed XAMPP).
- PDO Extension — Should be enabled by default if you’re using XAMPP, but if it’s not you’ll need to enable/install it.
1.3 File Structure & Setup
Navigate to C:\xampp\htdocs (XAMPP) and create the below directories and files.
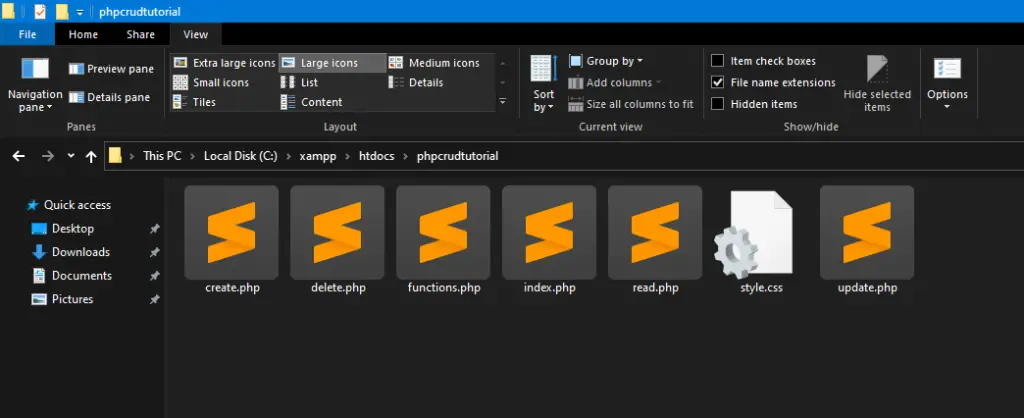
What will be in each file:
- index.php — Our CRUD app’s home page.
- create.php — Use an HTML form to create new records and a POST request to deliver data to the server.
- read.php — This file displays records from our database table and allows you to traverse through them using pagination.
- update.php — Use an HTML form to update existing records and a POST request to deliver data to the server.
- delete.php — Confirm and remove records based on their ID (GET request to get the ID).
- functions.php — Basic templating and MySQL connection routines (so we don’t have to repeat code in each file).
- style.css — This is the stylesheet for our app, and it will affect the way it looks.
Step 2. Crud Operations in PHP and MYSQL Database
In this step, we will be Creating the Database and setting up Tables for CRUD Operations in PHP.
We’ll store contacts in a MySQL database and retrieve them with PHP. If you’re using XAMPP, follow the steps below.
- Go to http://localhost/phpmyadmin/
- Under Create database, input phpcrud and select utf8_general_ci as the collation
- Create by clicking the Create button.
- Choose the recently created database.
- Select the SQL tab and run the following SQL:
CREATE TABLE IF NOT EXISTS `contacts` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL,
`email` varchar(255) NOT NULL,
`phone` varchar(255) NOT NULL,
`title` varchar(255) NOT NULL,
`created` datetime NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=13 DEFAULT CHARSET=utf8;
INSERT INTO `contacts` (`id`, `name`, `email`, `phone`, `title`, `created`) VALUES
(1, 'Jane Strong', '[email protected]', '2026550143', 'Lawyer', '2021-05-08 17:32:00'),
(2, 'Dwayne Johnson', '[email protected]', '2025550121', 'Employee', '2021-05-08 17:28:44'),
(3, 'Mitch Maypol', '[email protected]', '2004550121', 'Employee', '2021-05-08 17:29:27'),
(4, 'Jaggy Davidson', '[email protected]', '2022550178', 'Supervisor', '2021-05-08 17:29:27'),
(5, 'John Clover', '[email protected]', '7862342390', 'Janitor', '2021-05-09 19:16:00');
The above SQL will construct the contacts table, which we’ll utilize in our page. Also included in the SQL is sample data, which will be used for testing reasons to ensure everything is working well, and you may delete it afterwards.
The contacts database has six columns: id, name, email, phone, title, and created. The title column represents the role of each record; you may alter it to anything you like; the sample data will use work roles as an example.
In phpMyAdmin, the database should look like the following:
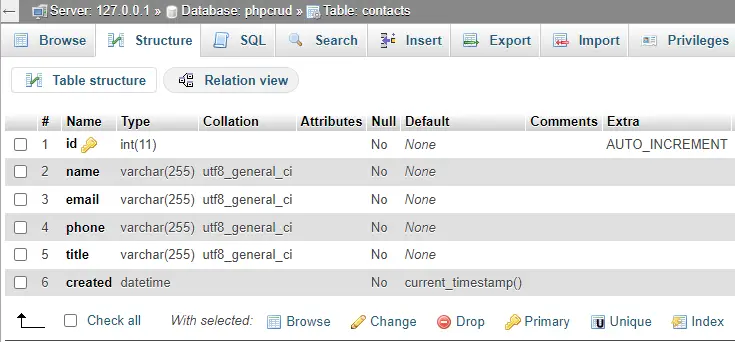
Step 3. Creating the CRUD Operations in PHP Stylesheet (CSS3)
The stylesheet will change the appearance of our CRUD Operations in PHP and MySQL app, edit the style.css file and add the following code:
* { box-sizing: border-box; font-family: -apple-system, BlinkMacSystemFont, "segoe ui", roboto, oxygen, ubuntu, cantarell, "fira sans", "droid sans", "helvetica neue", Arial, sans-serif; font-size: 16px; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; } body { background-color: #FFFFFF; margin: 0; } .navtop { background-color: #dd743a; height: 60px; width: 100%; border: 0; } .navtop div { display: flex; margin: 0 auto; width: 1000px; height: 100%; } .navtop div h1, .navtop div a { display: inline-flex; align-items: center; } .navtop div h1 { flex: 1; font-size: 24px; padding: 0; margin: 0; color: #fff; font-weight: normal; } .navtop div a { padding: 0 20px; text-decoration: none; color: #fff; font-weight: bold; } .navtop div a i { padding: 2px 8px 0 0; } .navtop div a:hover { color: #ffd8c2; } .content { width: 1000px; margin: 0 auto; } .content h2 { margin: 0; padding: 25px 0; font-size: 22px; border-bottom: 1px solid #ebebeb; color: #666666; } .read .create-contact { display: inline-block; text-decoration: none; background-color: #38b673; font-weight: bold; font-size: 14px; color: #FFFFFF; padding: 10px 15px; margin: 15px 0; } .read .create-contact:hover { background-color: #32a367; } .read .pagination { display: flex; justify-content: flex-end; } .read .pagination a { display: inline-block; text-decoration: none; background-color: #a5a7a9; font-weight: bold; color: #FFFFFF; padding: 5px 10px; margin: 15px 0 15px 5px; } .read .pagination a:hover { background-color: #999b9d; } .read table { width: 100%; padding-top: 30px; border-collapse: collapse; } .read table thead { background-color: #ebeef1; border-bottom: 1px solid #d3dae0; } .read table thead td { padding: 10px; font-weight: bold; color: #767779; font-size: 14px; } .read table tbody tr { border-bottom: 1px solid #d3dae0; } .read table tbody tr:nth-child(even) { background-color: #fbfcfc; } .read table tbody tr:hover { background-color: #376ab7; } .read table tbody tr:hover td { color: #FFFFFF; } .read table tbody tr:hover td:nth-child(1) { color: #FFFFFF; } .read table tbody tr td { padding: 10px; } .read table tbody tr td:nth-child(1) { color: #a5a7a9; } .read table tbody tr td.actions { padding: 8px; text-align: right; } .read table tbody tr td.actions .edit, .read table tbody tr td.actions .trash { display: inline-flex; text-align: right; text-decoration: none; color: #FFFFFF; padding: 10px 12px; } .read table tbody tr td.actions .trash { background-color: #b73737; } .read table tbody tr td.actions .trash:hover { background-color: #a33131; } .read table tbody tr td.actions .edit { background-color: #37afb7; } .read table tbody tr td.actions .edit:hover { background-color: #319ca3; } .update form { padding: 15px 0; display: flex; flex-flow: wrap; } .update form label { display: inline-flex; width: 400px; padding: 10px 0; margin-right: 25px; } .update form input { padding: 10px; width: 400px; margin-right: 25px; margin-bottom: 15px; border: 1px solid #cccccc; } .update form input[type="submit"] { display: block; background-color: #38b673; border: 0; font-weight: bold; font-size: 14px; color: #FFFFFF; cursor: pointer; width: 200px; margin-top: 15px; } .update form input[type="submit"]:hover { background-color: #32a367; } .delete .yesno { display: flex; } .delete .yesno a { display: inline-block; text-decoration: none; background-color: #38b673; font-weight: bold; color: #FFFFFF; padding: 10px 15px; margin: 15px 10px 15px 0; } .delete .yesno a:hover { background-color: #32a367; }
Change the design if you like, but this is what I came up with to make the CRUD app more attractive.
Step 4. Creating the CRUD Operations in PHP Page
Finally, we can begin coding the CRUD Operation in PHP Source Code Download. Make sure you’ve completed the preceding steps and have the MySQL database available before we begin.
4.1 Creating the Functions
This file will include functions that we can use in all of our PHP files, allowing us to avoid writing the same code in each one. After all, the less code we have to write, the better, right? We’ll construct three functions: one to connect to the database, and the other two to generate header and footer templates that will display on every page we make and include the HTML layout.
Add the following code to the functions.php file:
<?php function pdo_connect_mysql() { $DATABASE_HOST = 'localhost'; $DATABASE_USER = 'root'; $DATABASE_PASS = ''; $DATABASE_NAME = 'phpcrud'; try { return new PDO('mysql:host=' . $DATABASE_HOST . ';dbname=' . $DATABASE_NAME . ';charset=utf8', $DATABASE_USER, $DATABASE_PASS); } catch (PDOException $exception) { // If there is an error with the connection, stop the script and display the error. exit('Failed to connect to database!'); } } function template_header($title) { echo <<<EOT <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>$title</title> <link href="style.css" rel="stylesheet" type="text/css"> <link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.7.1/css/all.css"> </head> <body> <nav class="navtop"> <div> <h1>CRUD OPERATION IN PHP, PDO & MYSQL</h1> <a href="index.php"><i class="fas fa-home"></i>Home</a> <a href="read.php"><i class="fas fa-user"></i>Contacts</a> </div> </nav> EOT; } function template_footer() { echo <<<EOT </body> </html> EOT; } ?>
Make sure to replace the MySQL connection details with your own; we’ll be using PDO to connect to MySQL, and PDO will make it easier to communicate with our MySQL database.
4.2 Creating the Home Page
The index.php file will be served when you go to http://localhost/phpcrud/; this will be our home.
Add the following code to the index.php file:
<?php include 'functions.php'; ?> <?=template_header('Home')?> <div class="content"> <h2>Home</h2> <p>This is your home page</p> </div> <?=template_footer()?>
This will provide a simple home page from which we can browse to the other pages. As you can see, we include the functions.php file and run the template functions we developed, which will add the header and footer code to our home page.
If we go to http://localhost/phpcrud/ now, we’ll see something like this:
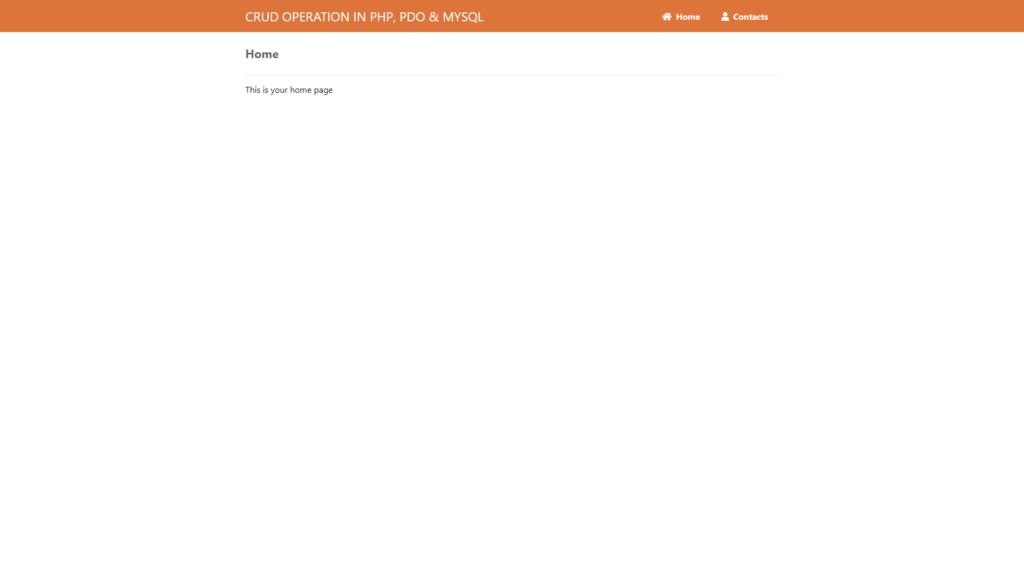
That’s pretty much all for the home; feel free to add your own material; this is only a portal to the rest of the site.
4.3 Creating the Read Page
This will create an HTML table with records from our table.
Add the following code to the read.php file:
<?php include 'functions.php'; // Connect to MySQL database $pdo = pdo_connect_mysql(); // Get the page via GET request (URL param: page), if non exists default the page to 1 $page = isset($_GET['page']) && is_numeric($_GET['page']) ? (int)$_GET['page'] : 1; // Number of records to show on each page $records_per_page = 5;
We include the functions file once more, but this time we use the function pdo connect mysql to connect to our MySQL database; if the connection is successful, we may utilize the $pdo
variable to perform queries.
We also create two more variables: the $page
variable will determine which page the user is currently on, and the $records_per_page
variable will be used to limit the number of records to display on each page. For example, if we limit the number of records to 5 and our contacts table has 10 records, there will only be 2 pages with 5 records on each page, and the user will be able to navigate between pages.
To the read.php file, add the following code:
// Prepare the SQL statement and get records from our table, LIMIT will determine the page $stmt = $pdo->prepare('SELECT * FROM contacts ORDER BY id LIMIT :current_page, :record_per_page'); $stmt->bindValue(':current_page', ($page-1)*$records_per_page, PDO::PARAM_INT); $stmt->bindValue(':record_per_page', $records_per_page, PDO::PARAM_INT); $stmt->execute(); // Fetch the records so we can display them in our template. $contacts = $stmt->fetchAll(PDO::FETCH_ASSOC);
The above code will choose data from the table, which will be decided by the user is on. The records will be arranged by the id column, but we can easily change it to created to sort the records by the create date instead.
For the aforementioned query, we’re also utilizing a prepared statement, which ensures that our query is safe (escapes user input data).
To the read.php file, add the following code:
// Get the total number of records, this is so we can determine whether there should be a next and previous button $num_contacts = $pdo->query('SELECT COUNT(*) FROM contacts')->fetchColumn(); ?>
Because the query does not involve user input variables, we don’t need to utilize a prepared statement in the above SQL query to acquire the total number of records in the table.
To the read.php file, add the following code:
<?=template_header('Read')?> <div class="content read"> <h2>Read Contacts</h2> <a href="create.php" class="create-contact">Create Contact</a> <table> <thead> <tr> <td>#</td> <td>Name</td> <td>Email</td> <td>Phone</td> <td>Title</td> <td>Created</td> <td></td> </tr> </thead> <tbody> <?php foreach ($contacts as $contact): ?> <tr> <td><?=$contact['id']?></td> <td><?=$contact['name']?></td> <td><?=$contact['email']?></td> <td><?=$contact['phone']?></td> <td><?=$contact['title']?></td> <td><?=$contact['created']?></td> <td class="actions"> <a href="update.php?id=<?=$contact['id']?>" class="edit"><i class="fas fa-pen fa-xs"></i></a> <a href="delete.php?id=<?=$contact['id']?>" class="trash"><i class="fas fa-trash fa-xs"></i></a> </td> </tr> <?php endforeach; ?> </tbody> </table> <div class="pagination"> <?php if ($page > 1): ?> <a href="read.php?page=<?=$page-1?>"><i class="fas fa-angle-double-left fa-sm"></i></a> <?php endif; ?> <?php if ($page*$records_per_page < $num_contacts): ?> <a href="read.php?page=<?=$page+1?>"><i class="fas fa-angle-double-right fa-sm"></i></a> <?php endif; ?> </div> </div> <?=template_footer()?>
This is the read template; the code iterates the contacts and adds them to the HTML table; when we browse to the read page, we’ll be able to read the entries in a table format.
Pagination has been added to the read page so that we may move between pages if you have more than 5 records (page 1, page 2, etc).
Font Awesome is used for the icons, therefore make sure it’s included in the header template function else the icons won’t show up.
Now, if we go to http://localhost/phpcrud/read.php, we can see the results. Here’s what we’ll see:
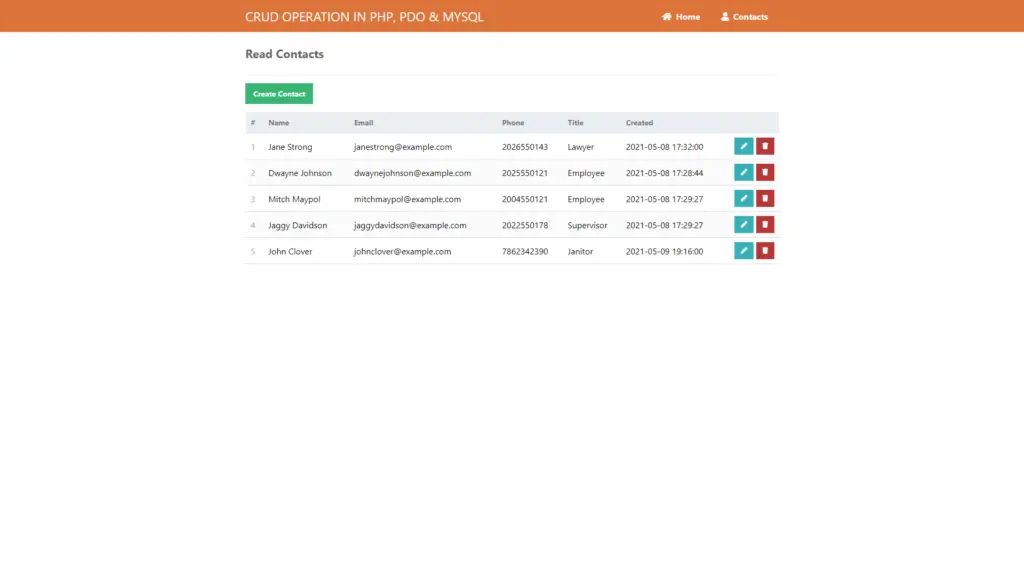
Now that we know how to show entries from our MySQL database, keep in mind that the buttons in the table (new, modify, and delete) will not operate since those pages have not yet been developed.
You may also get to the read by clicking the Contacts link in the top bar.
4.4 Creating the Create Page
New records will be created and inserted into our database using the create page.
Add the following to the create.php file:
<?php include 'functions.php'; $pdo = pdo_connect_mysql(); $msg = ''; // Check if POST data is not empty if (!empty($_POST)) { // Post data not empty insert a new record // Set-up the variables that are going to be inserted, we must check if the POST variables exist if not we can default them to blank $id = isset($_POST['id']) && !empty($_POST['id']) && $_POST['id'] != 'auto' ? $_POST['id'] : NULL; // Check if POST variable "name" exists, if not default the value to blank, basically the same for all variables $name = isset($_POST['name']) ? $_POST['name'] : ''; $email = isset($_POST['email']) ? $_POST['email'] : ''; $phone = isset($_POST['phone']) ? $_POST['phone'] : ''; $title = isset($_POST['title']) ? $_POST['title'] : ''; $created = isset($_POST['created']) ? $_POST['created'] : date('Y-m-d H:i:s'); // Insert new record into the contacts table $stmt = $pdo->prepare('INSERT INTO contacts VALUES (?, ?, ?, ?, ?, ?)'); $stmt->execute([$id, $name, $email, $phone, $title, $created]); // Output message $msg = 'Created Successfully!'; } ?>
The preceding code checks if the POST array (form data) is not empty; if it is, it signifies the user has completed the form and pressed the submit button, which will create a new entry in our table.
After that, add:
<?=template_header('Create')?> <div class="content update"> <h2>Create Contact</h2> <form action="create.php" method="post"> <label for="id">ID</label> <label for="name">Name</label> <input type="text" name="id" placeholder="26" value="Auto" id="id" readonly> <input type="text" name="name" placeholder="Cardo Dalisay" id="name" required> <label for="email">Email</label> <label for="phone">Phone</label> <input type="text" name="email" placeholder="[email protected]" id="email" required> <input type="text" name="phone" placeholder="2025550143" id="phone" required> <label for="title">Title</label> <label for="created">Created</label> <input type="text" name="title" placeholder="Employee" id="title" required> <input type="datetime-local" name="created" value="<?=date('Y-m-d\TH:i')?>" id="created" required> <input type="submit" value="Create"> </form> <?php if ($msg): ?> <p><?=$msg?></p> <?php endif; ?> </div> <?=template_footer()?>
This is the template for our create; as you can see, we’ve created a form and named each input field appropriately; the name of the input field is how we’ll get the POST variable in our PHP code; for example, if we name an input field “zip code,” we can get the value of that input field in PHP using $_POST['zip code']
(assuming the form’s method is set to post).
Now, if we go to http://localhost/phpcrud/create.php, we can see the results. We’ll see the following if we click the Create button on the read:
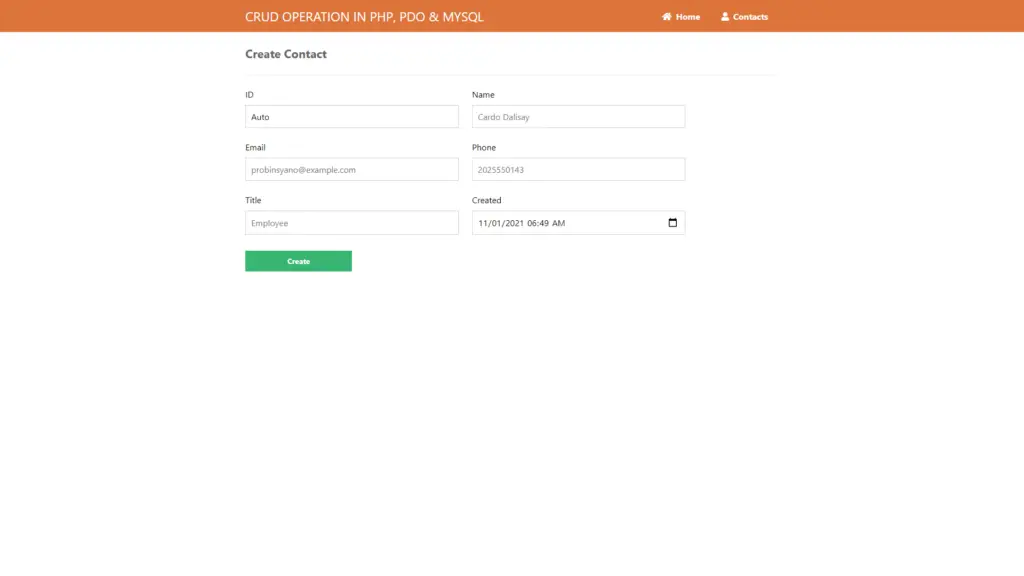
4.5 Creating the Update Page
The update will be used to edit records in our database; it is identical to the create page, except that instead of entering a new record, we will be changing existing records. With an Acquire request, we’ll be able to get the record ID.
Add the following to the update.php file:
<?php include 'functions.php'; $pdo = pdo_connect_mysql(); $msg = ''; // Check if the contact id exists, for example update.php?id=1 will get the contact with the id of 1 if (isset($_GET['id'])) { if (!empty($_POST)) { // This part is similar to the create.php, but instead we update a record and not insert $id = isset($_POST['id']) ? $_POST['id'] : NULL; $name = isset($_POST['name']) ? $_POST['name'] : ''; $email = isset($_POST['email']) ? $_POST['email'] : ''; $phone = isset($_POST['phone']) ? $_POST['phone'] : ''; $title = isset($_POST['title']) ? $_POST['title'] : ''; $created = isset($_POST['created']) ? $_POST['created'] : date('Y-m-d H:i:s'); // Update the record $stmt = $pdo->prepare('UPDATE contacts SET id = ?, name = ?, email = ?, phone = ?, title = ?, created = ? WHERE id = ?'); $stmt->execute([$id, $name, $email, $phone, $title, $created, $_GET['id']]); $msg = 'Updated Successfully!'; } $stmt = $pdo->prepare('SELECT * FROM contacts WHERE id = ?'); $stmt->execute([$_GET['id']]); $contact = $stmt->fetch(PDO::FETCH_ASSOC); if (!$contact) { exit('Contact doesn\'t exist with that ID!'); } } else { exit('No ID specified!'); } ?>
The above code will look for the contact ID, which will be a URL parameter, such as http://localhost/phpcrud/update.php?id=1. will return the contact with ID 1, and we can then process the request using the GET method and run a MySQL query to get the contact by ID.
After that, add:
<?=template_header('Read')?> <div class="content update"> <h2>Update Contact #<?=$contact['id']?></h2> <form action="update.php?id=<?=$contact['id']?>" method="post"> <label for="id">ID</label> <label for="name">Name</label> <input type="text" name="id" placeholder="1" value="<?=$contact['id']?>" id="id" readonly> <input type="text" name="name" placeholder="John Doe" value="<?=$contact['name']?>" id="name" required> <label for="email">Email</label> <label for="phone">Phone</label> <input type="text" name="email" placeholder="[email protected]" value="<?=$contact['email']?>" id="email" required> <input type="text" name="phone" placeholder="2025550143" value="<?=$contact['phone']?>" id="phone" required> <label for="title">Title</label> <label for="created">Created</label> <input type="text" name="title" placeholder="Employee" value="<?=$contact['title']?>" id="title" required> <input type="datetime-local" name="created" value="<?=date('Y-m-d\TH:i', strtotime($contact['created']))?>" id="created" required> <input type="submit" value="Update"> </form> <?php if ($msg): ?> <p><?=$msg?></p> <?php endif; ?> </div> <?=template_footer()?>
This is the update template; the input values are already defined in the contact fields, and the MySQL query we generated earlier will get them.
We should be able to click the update symbol next to a record on the read page and update it, and we should see something like this:
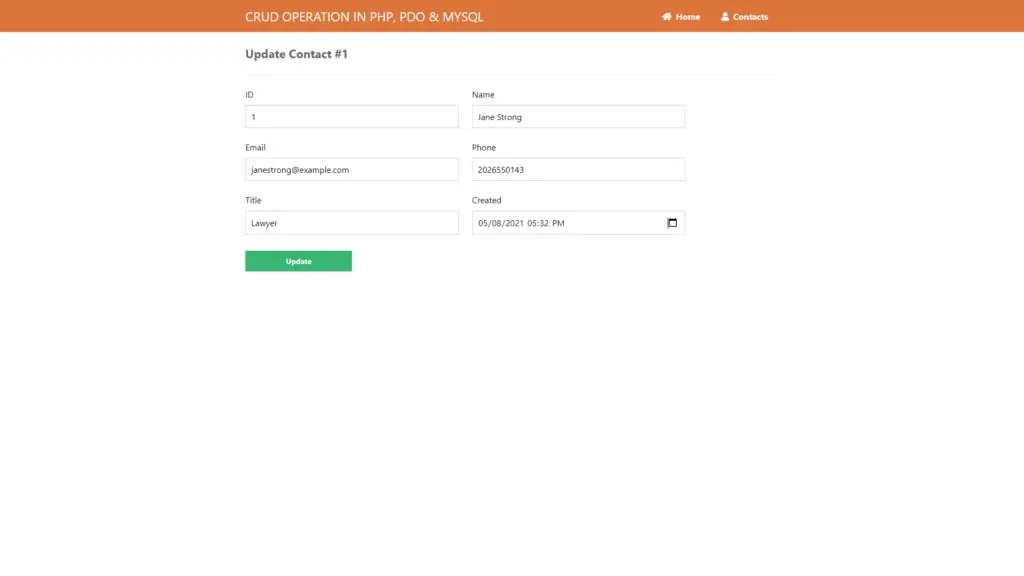
4.6 Creating the Delete Page
The table’s records will be deleted using the delete page. A user must confirm the deletion of a record before it can be deleted. This prevents inadvertent deletion.
Add the following to the delete.php file:
<?php include 'functions.php'; $pdo = pdo_connect_mysql(); $msg = ''; // Check that the contact ID exists if (isset($_GET['id'])) { // Select the record that is going to be deleted $stmt = $pdo->prepare('SELECT * FROM contacts WHERE id = ?'); $stmt->execute([$_GET['id']]); $contact = $stmt->fetch(PDO::FETCH_ASSOC); if (!$contact) { exit('Contact doesn\'t exist with that ID!'); } // Make sure the user confirms beore deletion if (isset($_GET['confirm'])) { if ($_GET['confirm'] == 'yes') { // User clicked the "Yes" button, delete record $stmt = $pdo->prepare('DELETE FROM contacts WHERE id = ?'); $stmt->execute([$_GET['id']]); $msg = 'You have deleted the contact!'; } else { // User clicked the "No" button, redirect them back to the read page header('Location: read.php'); exit; } } } else { exit('No ID specified!'); } ?>
To delete a record, the code will check whether the GET request variable “id” exists, if it does, then check if the record exists in the Contacts database and ask the user if they want to delete the contact or not (Yes or No).
After that, add:
<?=template_header('Delete')?> <div class="content delete"> <h2>Delete Contact #<?=$contact['id']?></h2> <?php if ($msg): ?> <p><?=$msg?></p> <?php else: ?> <p>Are you sure you want to delete contact #<?=$contact['id']?>?</p> <div class="yesno"> <a href="delete.php?id=<?=$contact['id']?>&confirm=yes">Yes</a> <a href="delete.php?id=<?=$contact['id']?>&confirm=no">No</a> </div> <?php endif; ?> </div> <?=template_footer()?>
The template for the delete is shown above, which includes the Yes and No buttons (delete confirmation) as well as the output message. The Yes and No buttons will launch a fresh GET request to confirm the user’s selection.
Click the delete button on one of the records on the read (Contacts), and you should see something like this:
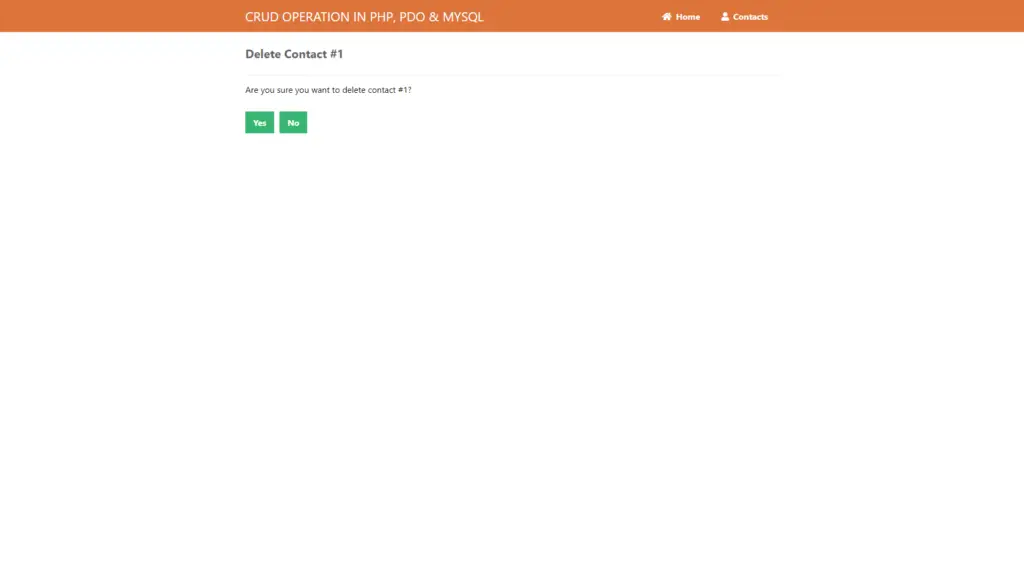
CRUD in PHP and MySQL with Source Code – Project Overview
Project Name: | CRUD Operation in PHP and MySQL with Source Code |
Abstract | The CRUD operations Create, Read, Update, and Delete table data. Because MySQL is widely used, understanding CRUD operations is critical for all developers. |
Language/s Used: | PHP |
PHP version (Recommended): | 5.6 or Newer |
Database: | MySQL |
Type: | Web Application, Website |
Developer: | IT Source Code |
Updates: | 0 |
Download CRUD System in PHP Source Code Below
Anyway, if you want to level up your programming knowledge, especially PHP, try this new article I’ve made for you Best PHP Projects With Source Code Free Download.
Conclusion
Congratulations! What happens now that you’ve constructed CRUD Operations in PHP and MySQL? Consider modifying the database and the code to include your own columns.
If you liked this tutorial, please share it using the social media buttons below and visit our website for more instructions.
And always remember that here in ITSourceCode, Source Code is not a problem.
Have fun coding!
Inquiries
If you have any questions or suggestions about CRUD Operation in PHP Source Code Download please feel free to contact me at our contact page or simply leave a comment below.
By the way, if you are interested in learning Java, I have here listed the Best Java tutorial for beginners with an example that can be used in practical ways.
You can try this Responsive Gallery using PHP CSS and Javascript