Filling Data in the Datagridview Based on ComboBox with SQL Server in C#
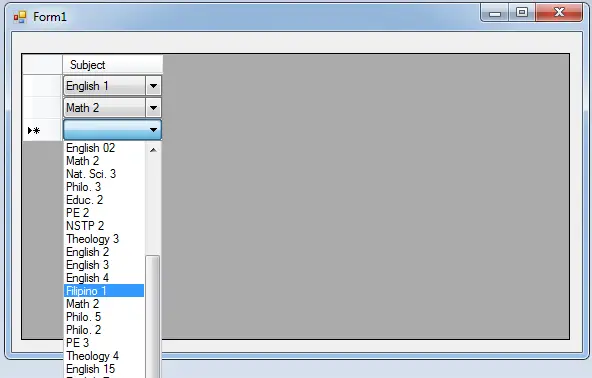
In this tutorial, I’m going to show you how to fill the data in the Datagridview based on Combobox using C# and SQL Server.
The process of this method is very simple, wherein you can easily retrieve the list of records that you have saved in the database and be loaded into the list control of a Combobox in the Datagriview.
These are the following steps:
note : For the database of this project just click here.
Step 1. Open Microsoft Visual Studio and create a new windows form application in C#. Then, drag a DataGridview on the form.
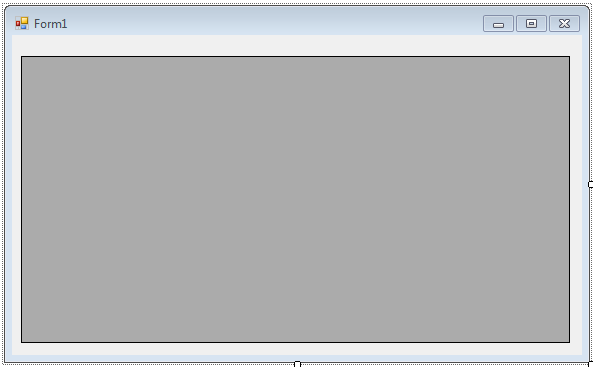
Step 2. Go to the solution explorer, click the “code view” to display the code editor.
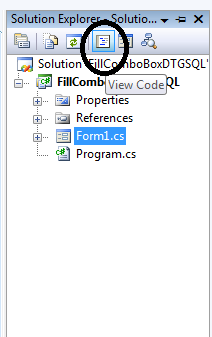
Step 3. Declare and initialize all classes that are needed.
//initializes new instance SqlConnection con = new SqlConnection(); SqlDataAdapter da = new SqlDataAdapter(); SqlCommand cmd = new SqlCommand(); DataTable dt = new DataTable();
Step 4. Create a method for filling data in a ComboBox.
private void LoadCombo() { //opening connection con.Open(); try { //initialize a new instance of sqlcommand cmd = new SqlCommand(); //set a connection used by this instance of sqlcommand cmd.Connection = con; //set the sql statement to execute at the data source cmd.CommandText = "SELECT * FROM tblsubject"; //initialize a new instance of sqlDataAdapter da = new SqlDataAdapter(); //set the sql statement or stored procedure to execute at the data source da.SelectCommand = cmd; //initialize a new instance of DataTable dt = new DataTable(); //add or resfresh rows in the certain range in the datatable to match those in the data source. da.Fill(dt); //initialize the combox column DataGridViewComboBoxColumn cbo = new DataGridViewComboBoxColumn(); //add data in the combobox foreach (DataRow r in dt.Rows) { //adding a list of records in the combobox cbo.Items.Add(r.Field<string>("SUBJCODE")); //name of combo box cbo.Name = "Subject"; } //adding combobox in the datagridview dataGridView1.Columns.Add(cbo); } catch (Exception ex) { //catching error MessageBox.Show(ex.Message); } //release all resources used by the component da.Dispose(); //closing connection con.Close(); }
Step 5. Go back to the design view, double-click the form and do the following codes for setting up the connection between SQL Server 2005 Express Edition and C#.Net. Then, call the “LoadCombo” method that you have created.
//set a connection between SQL server and Visual C# con.ConnectionString = "Data Source=.\\SQLEXPRESS;Database=dbsubject;trusted_connection=true;"; LoadCombo();
Output:
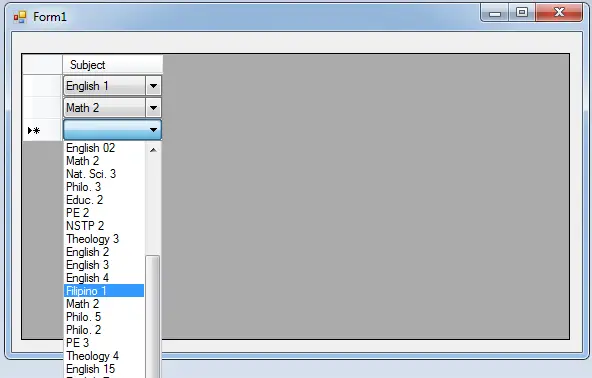
For all students who need a programmer for your thesis system or anyone who needs a source code in any a programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – TNT
ABOUT PROJECT | PROJECT DETAILS |
---|---|
Project Name : | Filling Data in the Datagridview Based on ComboBox with SQL Server |
Project Platform : | C# |
Programming Language Used: | C# Programming Language |
Developer Name : | itsourcecode.com |
IDE Tool (Recommended): | Visual Studio 2019 |
Project Type : | Desktop Application |
Database: | MySQL Database |
Upload Date and Time: | June 30, 2016- 5:28 am |