TOP OOPS INTERVIEW QUESTIONS C# 2023 – In this article, we’ll prepare you for the 2023 C# OOP Interview Questions.
Each interview is unique, but our list of top questions with answers will equip you to confidently handle your interview and boost your chances of success in your job hunt.
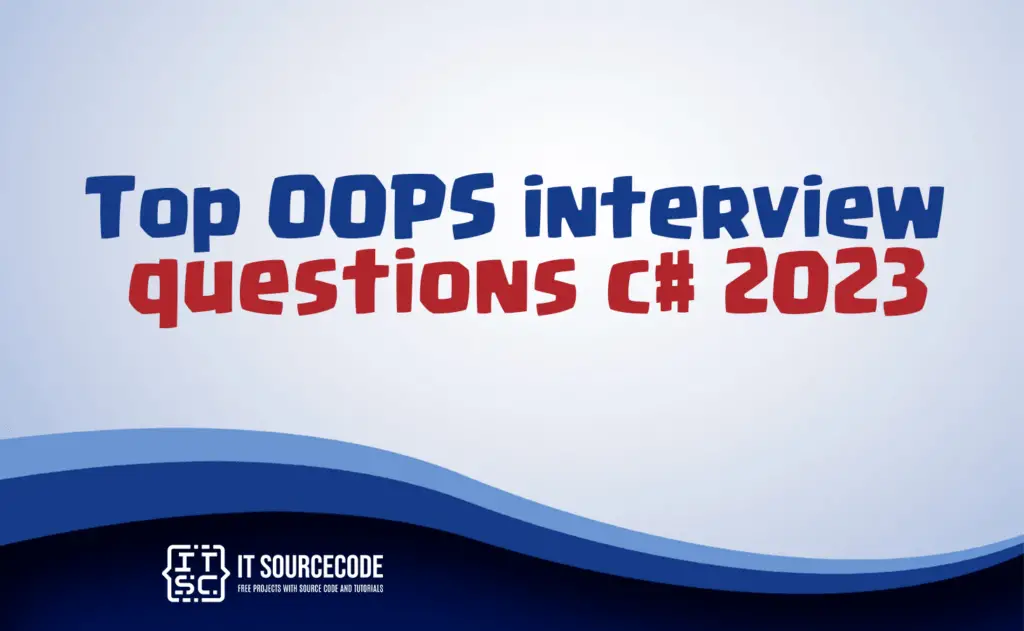
What is OOP?
Object-Oriented Programming (OOP) is a programming paradigm that organizes and structures code based on the concept of objects, which are instances of classes.
In OOP, data and behavior are encapsulated together within objects, promoting modularity, reusability, and ease of maintenance.
The four main principles of OOP are inheritance, encapsulation, polymorphism, and abstraction. Inheritance allows classes to inherit properties and behavior from other classes, encapsulation hides the internal implementation details of an object.
Polymorphism enables objects to take on multiple forms through method overriding and overloading, and abstraction allows the creation of simplified models to represent complex systems.
OOP fosters a more intuitive and human-like approach to programming, facilitating the development of scalable, efficient, and organized software systems.
Top OOPS interview questions c#
An abstract class can have both abstract and non-abstract methods, while an interface can only contain abstract methods.
In addition, a class can implement multiple interfaces, but it can inherit from just one abstract class. Moreover, an abstract class can include variables and constructors, which are not allowed in an interface.
Inheritance in C# is a foundational concept of object-oriented programming where a class (derived or child class) inherits properties and behaviors from another class (base or parent class).
The derived class can access and extend the functionalities of the base class, promoting code reuse and creating hierarchical relationships between classes.
Access modifiers in C# regulate the visibility and accessibility of class members (methods, properties, and fields).
The primary access modifiers include public, private, protected, internal, and protected internal. Public members are accessible from any code, while private members are limited to within the class.
Protected members are accessible within the class and its derived classes, and internal members are restricted to the same assembly. Lastly, protected internal members combine the accessibility of both protected and internal.
Polymorphism, a crucial concept in C# object-oriented programming, empowers objects to assume various forms.
C# facilitates two types of polymorphism: method overloading and method overriding. With method overloading, a class can possess multiple methods sharing the same name but varying parameters.
On the other hand, method overriding comes into play when a derived class offers a unique implementation for a method that already exists in its base class, utilizing the override keyword.
The sealed keyword is used to prevent further inheritance of a class.
Once a class is marked as sealed, it cannot serve as a base class for other classes, effectively disallowing any other class from inheriting from it.
This feature is employed to prevent further extension or modification of a specific class, promoting code stability and security.
Basic Question
Some distinctions between an Interface and an Abstract Class are as follows:
An abstract class can contain both abstract (unimplemented) and non-abstract (implemented) methods, whereas an interface can only have abstract methods.
Abstract classes can declare and use variables, but interfaces cannot have variables or fields.
In an abstract class, data members and methods are private by default, while in an interface, they are public and cannot be manually changed.
Abstract classes can have constructors, whereas interfaces cannot define constructors.
Delegates are reference-type variables in C# that store references to methods. They allow methods to be treated as objects, enabling dynamic invocation and runtime method selection.
Delegates provide a way to create type-safe function pointers, making it possible to pass methods as parameters to other methods or store them in data structures.
A delegate object can hold multiple references to methods in a sequence (Invocation List), which allows it to call multiple methods in the order they were added.
New functions can be added to this list at runtime using the += operator, and removal can be done using the -= operator. Delegates are commonly used in event handling and callback mechanisms.
Late binding and early binding refer to two different ways of linking or resolving method calls in a programming language.
Early Binding (Compile-time polymorphism) involves using methods with the same name but different parameter types or a different number of parameters.
This enables us to perform various tasks using the same method name within the same class, a concept known as method overloading.
On the other hand, Late Binding (Run-time polymorphism) occurs when the method calls are resolved during the program’s execution, rather than at compile-time.
This is typically achieved through the use of virtual and override keywords, enabling dynamic method dispatch and determining the method implementation at runtime based on the object’s actual type.
In scenarios where a class inherits multiple interfaces, and these interfaces have conflicting method names (i.e., methods with the same name but different signatures), the class cannot independently implement the conflicting methods due to the name and signature clashes.
To address this method conflict, we must use the explicit interface implementation approach. In this approach, the class implements the conflicting methods by explicitly mentioning the interface name before the method name.
This removes the ambiguity and allows the compiler to differentiate between the methods based on the interface they belong to.
By explicitly implementing the interfaces, the class ensures that the methods are only accessible through the corresponding interface references.
This way, we can resolve the method confusion that arises from inheriting multiple interfaces with conflicting method signatures.
Intermediate c# OOPs Interview Question
What is inheritance in C#?
Inheritance is a fundamental concept in object-oriented programming that allows a class (derived or child class) to inherit properties and behavior from another class (base or parent class).
This helps in achieving code reusability and creating a hierarchical relationship between classes.
What is the difference between method overriding and method overloading in C#?
Method Overriding: It is the ability of a derived class to provide a specific implementation for a method that is already defined in its base class.
The method signature (name, return type, and parameters) remains the same. The keyword used is override.
Method Overloading: It allows a class to have multiple methods with the same name but different parameters.
The methods should have different parameter types or a different number of parameters.
What is an abstract class in C#?
An abstract class is a special type of class that cannot be directly created or instantiated. Instead, it serves as a blueprint or foundation for other classes to derive from.
Within an abstract class, you can have both abstract methods, which are declared without any implementation, and non-abstract methods with complete implementations.
Derived classes that inherit from the abstract class must provide concrete implementations for all the abstract methods defined in the abstract class. The abstract class itself is identified by the “abstract” keyword in its declaration.
What are interfaces in C#?
An interface is a contract that defines a set of methods and properties that a class must implement if it implements the interface. It allows multiple inheritance in C# since a class can implement multiple interfaces.
Explain the sealed keyword in C#.
The sealed keyword is used to prevent further inheritance of a class. When a class is declared as sealed, it cannot be used as a base class for other classes, and no other class can inherit from it.
What is a static class in C#?
A static class is a class that cannot be instantiated, and all its members (methods and properties) must be static.
It is typically used to define utility classes or helper functions that do not require object state.
What is the purpose of the base keyword in C#?
The base keyword is used to access members of the base class from within a derived class.
It is particularly helpful when you want to call the base class’s constructor, access its methods, or properties from the derived class.
What are the access modifiers in C#?
C# has several access modifiers that control the visibility and accessibility of class members (methods, properties, fields) and classes. The main access modifiers are:
- public: The member or class is accessible from any code.
- private: The member is accessible only from within the same class.
- protected: The member is accessible within the same class and its derived classes.
- internal: The member or class is accessible within the same assembly (assembly-level access).
- protected internal: The member or class is accessible within the same assembly and its derived classes (combination of protected and internal).
Explain the concept of encapsulation in C#.
Encapsulation is one of the four fundamental principles of object-oriented programming (OOP). It involves bundling data (attributes) and methods (operations) that work on that data within a single unit (class).
The internal details of the class are hidden from the outside world, and interactions with the class are done through well-defined interfaces (properties and methods).
Encapsulation provides several benefits, including data security, code maintainability, and flexibility to change the internal implementation without affecting the outside code.
What is the difference between value types and reference types in C#?
Value Types:
- Value types are stored in the computer’s memory stack.
- They directly hold their data.
- When assigned to a new variable or passed to a method, a copy of the actual value is created.
- Examples of value types include int, float, char, bool, and struct.
Reference Types:
- Reference types are stored in the computer’s memory heap.
- They contain a reference or memory address pointing to the actual data stored in the heap.
- When assigned to a new variable or passed to a method, only the reference (memory address) is copied, not the entire data.
- Examples of reference types include class, interface, string, and array.
Advanced c# OOPs Interview Questions
Define accessibility modifiers and specify the number of them in C#.
Accessibility modifiers are keywords used in C# to determine the accessibility level of a member or type. In C#, there are five types of accessibility modifiers.
- Public: Allows unrestricted access to public members from any code.
- Private: Limits access to within the class where it is defined; if no modifier is specified, this is the default.
- Protected: Restricts access to the class where it is defined and any class that inherits from it.
- Internal: Limits access to classes defined within the current project assembly.
- Protected Internal: Allows access to classes defined within the current project assembly and its derived classes.
What is the purpose of a virtual method in C#?
A virtual method in C# allows developers to define a method in a base class that can be overridden (redefined) in derived classes.
The method in the base class is marked as virtual using the virtual keyword, and the derived class can provide its own implementation of the method using the override keyword.
This enables polymorphism, where the method called depends on the runtime type of the object rather than the reference type.
Explain what an extension method is in C#, and describe how to use it.
In C# object-oriented programming interviews, extension methods are often discussed. An extension method allows you to add new methods to existing types without creating a new derived type, modifying the original type, or recompiling the code.
To use an extension method, you need to import the namespace containing the extension methods into your source code using a directive.
Can the “this” keyword be used within a static method?
Since the “this” keyword refers to the current instance of a class, it cannot be used within a static method. Static members exist without any instance of the class and are called using the class name, not through an instance.
The “this” keyword is implicitly available in every constructor and non-static method, representing a reference variable of the class type in which it is defined.
How do I prepare for an OOP interview?
To get ready for an Object-Oriented Programming (OOP) interview, follow these steps:
- Understand OOP Concepts:
Grasp the fundamental principles of OOP, including inheritance, encapsulation, polymorphism, and abstraction. Gain practical knowledge of how these concepts are applied.
- Study C# Basics:
Familiarize yourself with C# programming language essentials, such as syntax, data types, variables, control structures, methods, and classes.
- Implement OOP in C#:
Practice applying OOP principles to create classes, inheritance hierarchies, and polymorphic behaviors in C# code. Work with abstract classes, interfaces, and method overriding.
- Learn Access Modifiers:
Comprehend the purpose of access modifiers in C# and how they control the visibility of class members. Practice using various modifiers like public, private, protected, internal, and protected internal.
- Master Polymorphism
Practice method overloading and method overriding in C#. Grasp how polymorphism allows objects to take on different forms.
- Explore Design Patterns:
Study common design patterns like Singleton, Factory, and Observer, and understand how they are implemented in C#.
- Solve OOP Problems
Enhance problem-solving skills by tackling OOP-related coding exercises and challenges.
- Understand Inheritance
Gain proficiency in implementing inheritance in C# to create class hierarchies and understand how derived classes inherit properties and behaviors.
- Embrace OOP Best Practices
Learn and apply OOP best practices, such as cohesion, loose coupling, and the Single Responsibility Principle, to write clean and maintainable code.
- Review Past Projects
Reflect on past OOP projects, examining challenges faced and the solutions employed.
- Study OOP Interview Questions:
Review common OOP interview questions and ensure you understand the underlying concepts behind them.
- Conduct Mock Interviews:
Simulate real interview scenarios by conducting mock interviews with a friend or mentor.
- Stay Updated:
Keep abreast of the latest trends and advancements in OOP and C#. Follow industry blogs, articles, and online resources
- Prepare Questions:
Prepare insightful questions to ask the interviewer about the company’s OOP practices and projects during the interview.
- Exude Confidence:
Stay composed and confident during the interview, effectively communicating your knowledge of OOP and its practical applications
By following these steps, you will be well-prepared to face any OOP interview confidently, showcasing your expertise in C# and OOP concepts.
Additional Resources
- Exploring Microsoft Excel Jobs Opportunities
- Top OOPS Interview Questions C# 2023
- Exploring Microsoft Excel Jobs Opportunities
Conclusion
Embarking on your path as a coder? Rather than trying to grasp everything simultaneously, it’s advisable to proceed gradually.
Kickstart your journey today by exploring concise courses such as Full Stack Web Developer – MEAN Stack and AWS Cloud Architect. These offerings will sharpen your essential skills and swiftly prepare you for the job market.