In this tutorial, I will teach you how to connect MySQL database in c# windows application using Microsoft Visual Studio 2008. With this, you can establish a connection between MySQL Database and C#.net.
About Project Details
ABOUT PROJECT | PROJECT DETAILS |
---|---|
Project Name : | How to Connect MySQL to Database in C# |
Project Platform : | C# |
Programming Language Used: | C# Programming Language |
Developer Name : | itsourcecode.com |
IDE Tool (Recommended): | Visual Studio 2019 |
Project Type : | Desktop Application |
Database: | MYSQL DATABASE |
Can you connect C# to MySQL?
A MySqlConnection Object serves as the intermediary for all of the communication that takes place between a MySQL server and a C# application.
In order for your application to be able to communicate with the server, it first needs to create an instance of the MySqlConnection object, configure it, and then open it.
What is SQL connection in C#?
A SqlConnection object represents a unique session to a SQL Server data source. When using a client-server database architecture, this function is analogous to establishing a network connection to the server.
When connecting to a Microsoft SQL Server database, the efficiency of the connection can be improved by utilizing SqlConnection in conjunction with SqlDataAdapter and SqlCommand.
Can you use SQL in C#?
C# has the ability to execute a select command written in SQL against a database. The ‘SQL’ statement can be utilized in order to retrieve data from a certain table within the database.
The process of inserting data into the database can also be accomplished with C#, which can be used to add records to the database.
Let’s begin:
1. Create a database in the MySQL and name it “dbconnect“
2. Open Microsoft Visual Studio and create a new windows form application for C#. Then, drag a button and do the form just like this.
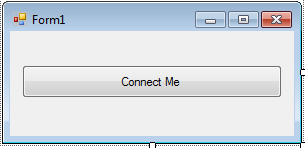
3. Double-click a button to fire the click event handler of it.
4. After that, do the following codes to established a connection between MySQL Database and C#.net in the method.
Note: Add “MySQL.Data.dll” as your refernce and put “using MySql.Data.MySqlClient;“ above the namespace to access MySQL library.
//initialize sql connection MySqlConnection con = new MySqlConnection(); //set a connection string con.ConnectionString = "server=localhost;user id=root;password=;Database=dbconnect;"; //opening connection con.Open(); //validating connection if (con.State == ConnectionState.Open) { if (button1.Text == "Connect Me") { button1.Text = "Disconnect Me"; this.Text = "Connected"; } else { button1.Text = "Connect Me"; this.Text = "Disconnected"; con.Close(); } }
5. Press F5 on the keyboard to run your project.
Output:
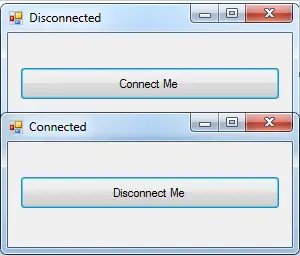
This is a simple yet powerful method to do because without this, you can’t proceed to the process of CRUD.